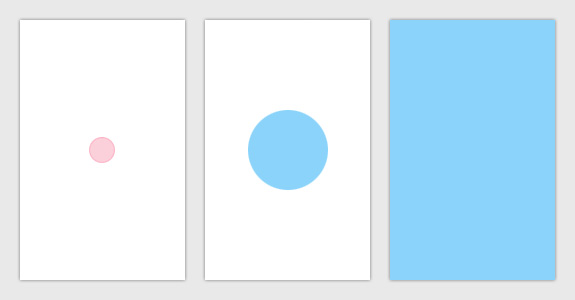
As a bit of an experiment for this site I wanted to mimic the confirmation of click/touch that Material design allows on apps.
Essentially its a circular colour that radiates out from where you touch to show the user that an action has taken place.
Here’s the basic structure of my links:
$('.link').on('click', function(){
$(this).find('.circle').addClass('runcircle');
var href = $(this).find('.mixhover').attr('href');
setTimeout(function() {window.location = href}, 450);
return false;
});
I have a little bit of jQuery where it adds a class to the circle element (the added class has a CSS animation attached to it) and adds a timer to the actual link so the animation has time to run.
$('.link').on('click', function(){
$(this).find('.circle').addClass('runcircle');
var href = $(this).find('.mixhover').attr('href');
setTimeout( function() {window.location = href}, 450);
return false;
});
The circle span has the following CSS:
.circle {
position: absolute;
top: calc(50% - 5px);
left: calc(50% - 5px);
width: 10px;
height: 10px;
background: #4285F4;
border-radius: 10px;
opacity: 0;
z-index: 1;
}
… and when the runcircle class is added it does (width and height have to be bigger than the container its in to work):
.circle.runcircle {
top:-200px;
left:-200px;
width:600px;
height:600px;
border-radius:500px;
animation-name: circlefade;
animation-duration: .4s;
animation-timing-function:
linear;
}
The css animation is as follows:
@keyframes circlefade {
0% { opacity:0; }
10% { opacity:.5; }
20% { opacity:.5; }
30% { opacity:.5; }
40% { opacity:.5; }
50% { opacity:.5; }
60% { opacity:.45; }
70% { opacity:.35; }
80% { opacity:.25; }
90% { opacity:.15; }
100% { opacity:0; }
}
Then as a bonus if you want to test the animation over and over to get it how you want, comment out (or remove) the window.location line so the ‘return false’ stops the link from working.
Then you can add this to remove the ‘runcircle’ class so you can see your animation, then click it again and again and again…
$(".link .circle").on("webkitAnimationEnd oanimationend msAnimationEnd animationend",function() {
$(this).delay(200).queue(function() {
$(this).removeClass("large").dequeue();
});
});
So if all goes to plan you should get a nice circle animation from the center of the container out.